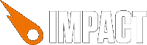
Animations in Impact are handled with an AnimationSheet – a big image containing all individual animation frames.
The frame numbering of an AnimationSheet starts at
0
in the upper left corner and continues in reading direction. Note that this is different from the tile numbering for BackgroundMaps, which starts at 1
.
To create an AnimationSheet, you specify the image file to use, as well as the width and height for each frame in this image. This AnimationSheet should be created as a class property to be loaded by the preloader. See Working With Assets for more info.
When you can create a new Animation, you hand over the AnimationSheet, the time each frame is displayed in seconds and an array of frames, specifying the sequence.
// Create an AnimationSheet with the blob.png file. // Each frame is 16x16 px var animSheet = new ig.AnimationSheet( 'blob.png', 16, 16 ); // Create an animation using the AnimationSheet. // Display each frame for 0.2 Seconds. The Sequence // is [2,3,4] and the Animation stops at the last // frame (last parameter is true) var jumpAnim = new ig.Animation( animSheet, 0.2, [2,3,4], true );
After you have created an Animation, you have to update it for each game frame using .update()
and you can draw it using .draw()
.
When animating Entities you can use some of the entity's utility methods to make your life a bit easier.
Each entity has an .animSheet property, where you can load the default AnimationSheet for it.
Entities also have an .anims object, to which all the animations you define with the .addAnim() method are added to. The .addAnim()
method takes the same parameters like the constructor for Animations, except that it always uses the .animSheet
as the AnimationSheet.
The entitie's .draw() method automatically draws the .currentAnim at the entities position.
// Create your own entity, subclassed from ig.Enitity EntityBlob = ig.Entity.extend({ // Load an animation sheet animSheet: new ig.AnimationSheet( 'blob.png', 16, 16 ), init: function( x, y, settings ) { // Add animations for the animation sheet this.addAnim( 'idle', 1.5, [1,1,2] ); this.addAnim( 'jump', 0.2, [2,3,4], true ); // Call the parent constructor this.parent( x, y, settings ); } });
This entity can already be used in Weltmeister and will be animated in the game. It's .currentAnim
will be set to the 'idle'
Animation, because it was added first.
To switch between animations, you can just set .currentAnim
to one of the Animations now defined in .anims
. E.g.:
update: function() { if( someCondition ) { this.currentAnim = this.anims.jump.rewind(); } else { this.currentAnim = this.anims.idle; } }
For BackgroundMaps you can specify certain tiles to be animated. Each BackgroundMap has an .anims property that maps a tile number to an Animation. E.g.:
var data = [ [1,2,6], [0,3,5], [2,8,1], ]; var bg = new ig.BackgroundMap( 16, data, 'media/tileset.png' ); // The image used for the AnimationSheet *can* be different from // the one used as the tileset for the BackgroundMap var as = new ig.AnimationSheet( 'media/tiles.png', 16, 16 ); // Sets tile number 5 of the BackgroundMap to be animated bg.anims[5] = new ig.Animation( as, 0.1, [0,1,2,3,4] );
Note that Animations are not updated by the BackgroundMap, but must be updated externally. ig.Game has some facilities for that. See .backgroundAnims.