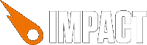
The ImagePicker class allows you to load Images for drawing in Canvas2D oder WebGL from the iOS Camera Roll
var imagePicker = new Ejecta.ImagePicker(); imagePicker.getPicture(function (error, image) { if (error) { return console.log('Loading failed: ' + error); } ctx.drawImage(image, 0, 0); });
Opens the Image Picker and upon completion calls the callback with an image or an error string. The options
argument can be used to configure certain aspects of the Image Picker. See the example below for the details.
Complete example:
var canvas = document.getElementById('canvas'); var w = canvas.width = window.innerWidth; var h = canvas.height = window.innerHeight; var ctx = canvas.getContext('2d'); ctx.fillStyle = '#000000'; ctx.fillRect(0, 0, w, h); ctx.fillStyle = "#ffffff"; ctx.fillText('Tap the screen to open the photo library', 0, 20); document.addEventListener('touchend', function (ev) { var imagePicker = new Ejecta.ImagePicker(); // all options are optional var options = { sourceType : 'PhotoLibrary', // 'PhotoLibrary' or 'SavedPhotosAlbum' (or experimental 'Camera') popupX : ev.changedTouches[0].pageX, // Popup position, relevant only on iPad when sourceType is not Camera, popupY : ev.changedTouches[0].pageY, // best value is the tap coordinates or the center of the tapped element maxWidth : w, // Max image size (resolution), by default it's GL ES max texture size maxHeight : h // .. }; // Setting the 'sourceType' to 'camera' is experimental because: // - an iPad app in portrait mode only presents the camera interface in landscape no matter what // - it have not been tested on a large enough number of different devices / iOS versions // - internet seems to report more strange camera orientation behaviors depending of the device / iOS version // The right way to access and retrieve a picture from the camera seems to be the usage of ObjC's AVCam library // instead of UIImagePicker, and would require another specific EJbinding oriented on the camera. // This beeing said, this option is here because it works well in some particular cases (like an iPhone's portrait // only application) and because Ejecta doesn't provide yet any another way to access the camera. But if you are // using it, it's at your own risk :). // Note: on iPhone the image picker is displayed on portrait mode only. This is an Apple requirement: // "The UIImagePickerController class supports portrait mode only. This class is intended to be used as-is and // does not support subclassing." // There is some hacks around to override this behavior, but because Apple may reject your app if you do this // and because those hacks are not easy to implement on all iOS/devices, they are not available in this EJbinding. imagePicker.getPicture(function (error, image) { if (error) { return console.log('Loading failed: ' + error); } ctx.drawImage(image, 0, 0); }, options); }, false);