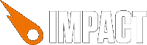
This class implements In App Purchases for Apples App Store. Note that you have to set up all products in iTunesConnect first – with the correct bundle identifier as specified in your in Info.plist
.
var iap = new Ejecta.IAPManager(); // Request the 'shotgun' and 'plasmagun' products iap.getProducts( ['shotgun', 'plasmagun'], function(error, products) { if( error ) { console.log( error ); } else { // Log all product info and search for the shotgun var shotgun = null; for( var i = 0; i < products.length; i++ ) { console.log( products[i].id, products[i].title, products[i].description, products[i].price ); if( products[i].id == 'shotgun' ) { shotgun = products[i]; } } // Buy 1 shotgun shotgun.purchase(1, function(error, transaction) { if( error ) { console.log(error); } else { // Purchase successful; log some transaction info console.log( transaction.productId, transaction.id, transaction.receipt ); } }); } }); // Call this to restore all transaction - i.e. when the user re-installs // your app on a new device iap.restoreTransactions(function(error, transactions) { if( error ) { console.log( error ); } else { for( var i = 0; i < transactions.length; i++ ) { console.log( transactions[i].productId, transactions[i].id, transactions[i].receipt ); } } });
Load the products with the given products IDs.
The supplied callback function is called with an error
parameter that is either null
or a localized error string. On success, the second parameter is an array of IAPProducts
.
Restores all transactions for the current User account. Apple requires this option to be present in your App. The user should be able to call this function (e.g. through a menu button) when he re-installs the App on his device.
The supplied callback function is called with an error
parameter that is either null
or a localized error string. On success, the seccond parameter is an array of IAPTransactions
.
The localized description of the product, as specified in iTunesConnect.
The ID name of the product, as specified in iTunesConnect.
The localized price string, e.g. $0.99 or 0.79€.
Purchases the given quantity of the IAPProduct.
The supplied callback function is called with an error
parameter that is either null
or a localized error string.
On success, the seccond parameter is an instance of IAPTransaction
.
The unique ID of this transaction
The product ID associated with this transaction
The transaction's receipt as Base64 encoded string.