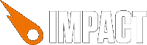
getContext(type, [options])
- supports '2d'
and 'webgl'
('experimental-webgl'
) types. The optional 2nd parameter accepts an object like {antialias: true, antialiasSamples: 4}
.toDataURL()
- supports image/png
and image/jpeg
width
height
style
- .width
, .height
, .left
, .top
save()
restore()
scale(x, y)
rotate(angle)
translate(x, y)
transform(m11, m12, m21, m22, dx, dy)
setTransform(m11, m12, m21, m22, dx, dy)
globalAlpha
globalCompositeOperation
supports source-over
, lighter
, darker
, destination-out
, destination-over
, source-atop
, xor
, copy
, source-in
, destination-in
, source-out
, destination-atop
blend modesstrokeStyle
fillStyle
lineWidth
lineCap
lineJoin
miterLimit
clearRect(x, y, w, h)
fillRect(x, y, w, h)
strokeRect(x, y, w, h)
beginPath()
closePath()
moveTo(x, y)
lineTo(x, y)
quadraticCurveTo(cpx, cpy, x, y)
bezierCurveTo(cp1x, cp1y, cp2x, cp2y, x, y)
arcTo(x1, y1, x2, y2, radius)
rect(x, y, w, h)
arc(x, y, radius, startAngle, endAngle, anticlockwise)
fill( [fillRule] )
- supports non-zero
and even-odd
fill rulesstroke()
createLinearGradient(x0, y0, x1, y1)
createRadialGradient(x0, y0, r0, x1, y1, r1)
createPattern(image, repetition)
clip()
- note: has problems with restore(); it only restores the last clip pathdrawImage(image, dx, dy)
drawImage(image, dx, dy, dw, dh)
drawImage(image, sx, sy, sw, sh, dx, dy, dw, dh)
getImageData(sx, sy, sw, sh)
putImageData(data, dx, dy)
getImageDataHD(sx, sy, sw, sh)
putImageDataHD(data, dx, dy)
createImageData(sw, sh)
imageSmoothingEnabled
font
textBaseline
textAlign
fillText(text, x, y)
strokeText(text, x, y)
isPointInPath(x, y)
and shadows are not yet implemented.
font
is quite picky at what it accepts. The format must be XXpt Name
, e.g.:
ctx.font = '10pt Zapfino';
To load custom, non-system fonts use ejecta.loadFont()
ejecta.loadFont('my-font.tff');
Ejecta fully implements the WebGL 1.0 Spec.
play()
pause()
load()
canPlayType()
supports audio/x-caf
, audio/mpeg
and audio/mp4
cloneNode()
canplaythrough event
ended event
– only for large sound files (>512kb)loop
volume
currentTime
src
preload
playbackRate
– accepts values between 0.5 and 2ended
muted
paused
ended
src
currentTime
controls
loop
volume
(currently does nothing)duration
canPlayType(type)
supports 'video/mp4'load()
play()
pause()
loadedmeta
, canplaythrough
, ended
and click
eventsExample:
var video = document.createElement('video'); console.log( 'can play mp4', video.canPlayType('video/mp4') ); video.src = 'test.mov'; // Also loads from the internet: //video.src = 'http://km.support.apple.com/library/APPLE/APPLECARE_ALLGEOS/HT1211/sample_iTunes.mov'; // Show playback controls: //video.controls = true; // For local video files, you don't really have to wait for the // 'canplaythrough' event you can just call .play() after setting the .src video.addEventListener('canplaythrough', function(){ console.log('duration', video.duration); video.play(); // Jump to 100 seconds: //video.currentTime = 100; video.addEventListener('click', function(){ video.pause(); }, false); video.addEventListener('ended', function(){ console.log('ended!'); }, false); }, false);
src
width
height
complete
load
and error
eventsEjecta supports loading of .jpg, .png and .pvr (PVRTC compressed) Images.
getItem(key)
setItem(key, value)
removeItem(key)
clear()
key()
length
Note that getItem()
and setItem()
only accept string values and keys, but you can easily save objects with JSON.stringify(obj)
and retrieve them with JSON.parse(string)
again.
createElement()
supports image
, canvas
, audio
getElementById()
only accepts canvas
; returns the screen canvastouchstart
, touchmove
and touchend
eventsdevicemotion
and deviceorientation
eventhidden
visibilityState
pagehide
, pageshow
and visibilitychange
eventsresize
eventThe whole document
object is a thin, "fake" layer, meant to provide some common behavior for loading additional script files and handling touch and devicemotion events. E.g. the following examples will work in Ejecta as well as in a normal Browser:
// Get the main screen canvas var canvas = document.getElementById('canvas'); // Create a second, off-screen canvas var offscreen = document.createElement('canvas'); // Load an additional script file asynchronously var script = document.createElement('script'); script.src = 'some-source-file.js'; document.body.appendChild(script); // Log coordinates for touchmove event document.addEventListener( 'touchmove', function( ev ) { console.log( ev.touches[0].pageX, ev.touches[0].pageY ); }, false ); // Log accelerometer data document.addEventListener( 'devicemotion', function( ev ) { var accel = ev.accelerationIncludingGravity; console.log( accel.x, accel.y, accel.z ); }, false );
See Other Sources/ejecta.js
if you want to implement additional default behavior.
log(message, ...)
– logs objects up to 2 levels deep, abbreviates arrayslogJSON(message, ...)
– logs objects all the way downdebug(message, ...)
info(message, ...)
warn(message, ...)
error(message, ...)
assert(assertion, message, ...)
time(name)
timeEnd(name)
devicePixelRatio
innerWidth
innerHeight
screen.availWidth
screen.availHeight
navigator.userAgent
reports iPhone
or iPad
navigator.appVersion
navigator.platform
navigator.onLine
navigator.getGamepads()
open(url)
– opens the given URL in Mobile SafarisetTimeout(callback, timeout)
clearTimeout(timeoutId)
setInterval(callback, interval)
clearInterval(intervalId)
requestAnimationFrame(callback)
cancelAnimationFrame(id)
performance.now()
orientation
Supports Gamepads on iOS and tvOS. It closely follows the W3C Gamepad API Spec – including the "standard" mapping.
On tvOS, the TV Remote is mapped to the appropriate buttons, the touchpad is mapped to an analog stick and(!) a D-Pad. The one addition to the W3C spec is the gamepad.exitOnMenuPress
flag. See here for the fascinating details about why this flag exists.
For a full example see this comment.
PERMISSION_DENIED
, POSITION_UNAVAILABLE
, TIMEOUT
constantswatchPosition( callback, [errback] )
clearWatch(id)
getCurrentPosition( callback, [errback], [options] )
Example:
var success = function(position) { console.log('position', JSON.stringify(position.coords), position.timestamp); }; var error = function(error) { if( error.code === navigator.geolocation.PERMISSION_DENIED ) { // Remember that we don't have permissions and don't try again } console.log('error', error.code, error.message); } // One shot location - aims to return fast and may be imprecise. // Set enableHighAccuracy to true for better results navigator.geolocation.getCurrentPosition(success, error, {enableHighAccuracy:false});
More over at github.
send( message )
send String or TypedArrayreadyState
url
– read onlybufferedAmount
(always 0)protocol
extensions
binaryType
(only supports "arraybuffer"
)CONNECTING
, OPEN
, CLOSING
, CLOSED
constants for .readyState
message
, open
, error
and close
eventsExample:
var socket = new WebSocket('ws://example.com:8787') socket.addEventListener('open', function() { console.log('open'); socket.send('hello'); }); socket.addEventListener('message', function(event) { console.log(event.data) }); socket.addEventListener('close', function() { console.log('close'); }); socket.addEventListener('error', function(error) { console.log(error) });
open(method, url, [async], [user], [pass])
send(data)
setRequestHeader(header,value)
abort()
getAllResponseHeaders()
getResponseHeade(header)
overrideMimeType(type)
readyState
responseType
("text"
, "json"
or "arraybuffer"
)response
(text or JSON object, depending on responseType
)responseText
statusText
status
timeout
UNSENT
, OPENED
, HEADERS_RECEIVED
, LOADING
and DONE
constants for .readyState
readystatechange
loadend
, load
, error
, abort
, progress
, loadstart
and timeout
eventsExample:
var request = new XMLHttpRequest(); request.onreadystatechange = function() { if( request.readyState == request.DONE && request.status == 200 ) { console.log( 'server', request.getResponseHeader('server') ); console.log( request.responseText ); } }; request.open('GET', 'http://www.google.com/'); request.send();
As of Ejecta 1.2, XMLHttpRequest can also be used to load local files. The file path is relative to the App/
directory.
Use req.overrideMimeType('text/plain; charset=x-user-defined');
to load binary data as text. The newer, better way of loading binary data is to set the responseType
to 'arraybuffer'
include(file)
openURL(url, [message])
getText(title, message, callback)
loadFont(path)
allowSleepMode
- boolean if the device is allowed to sleep (default false
)audioSession
– "ambient"
, "solo-ambient"
or "playback"
(see AVAudioSession Categories)// This loads an additional script file synchronously (blocking) ejecta.include('some-source-file.js'); // Open a URL in Mobile Safari ejecta.openURL( 'http://impactjs.com/', 'Open the Impact website?' ); // Ask the user for some text: ejecta.getText( 'Highscore', 'Please enter your name', function(text) { console.log(text); }); // CommonJS style require var inc = require('math/basics').increment; // Load a custom TTF font, similar to a CSS @font-face declaration ejecta.loadFont("path/to/my/font.ttf");