1 decade ago
by jul
I've created a little maze game similar to Pacman in order to learn more about the engine, but have run into a bit of random behaviour and thought I'd ask for advice.
It's probably best explained with a picture so I've attached one below and changed the sprite to a simple square for debugging purposes. The white tiles are linked to the collision map and the gaps are exactly 64x64 so that the entity which is also 64x64 should be able to go through.
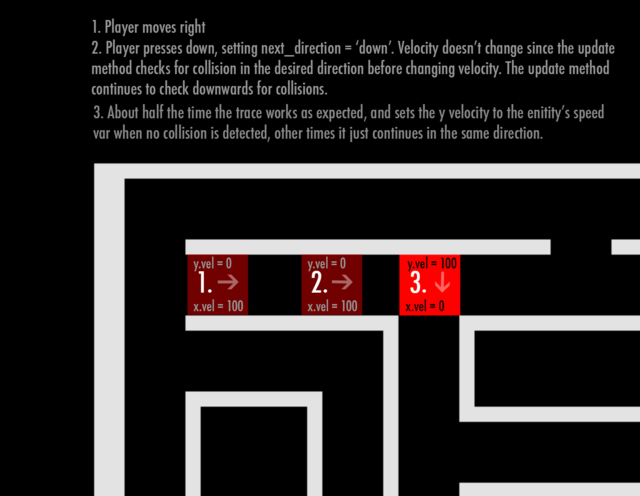
//make sure the entity's positions are integers
this.pos.x = Math.floor(this.pos.x);
this.pos.y = Math.floor(this.pos.y);
if (this.direction_next === 'down') {
var trace = ig.game.collisionMap.trace(this.pos.x, this.pos.y, 0, 1, this.size.x, this.size.y);
if (!trace.collision.y) {
this.vel.y = +this.speed;
this.vel.x = 0;
}
}
Any ideas what I'm doing wrong here or a better way to solve it would be highly appreciated. If I change the entity's speed to something super slow it always works as intended so maybe I need a more efficient way to detect the gaps in the maze.
Cheers
I've seen a problem that may be related to yours, whereby calculations that should return say, 128, would instead return 127.999999. Solved by rounding whenever precision of calcs, as in your case, is needed.
1 decade ago
by Xatruch
Hi, maybe it's not a trace problem but an update problem. Maybe the entity doesn't check if (!trace.collision.y) properly at high speed as you said.
try a different location of the code.
//make sure the entity's positions are integers
this.pos.x = Math.floor(this.pos.x);
this.pos.y = Math.floor(this.pos.y);
var trace = ig.game.collisionMap.trace(this.pos.x, this.pos.y, 0, 1, this.size.x, this.size.y);
if (this.direction_next === 'down' && !trace.collision.y) {
this.vel.y = +this.speed;
this.vel.x = 0;
}
1 decade ago
by jul
Hi Xatruch yeah it looks like it's an update problem. After debugging some more I can see that the gap lines up with the player when player's pos.x is exactly 320. Problem is that update method isn't always called frequently enough so that the player is at exactly x=320. Sometimes I get movement logs (pos.x) like [314, 315, 317, 322, 323] or similar meaning the trace actually returns correctly and the player is never really at the exact spot of the gap.
I'm running this on a new Macbook pro retina so I would imagine the accuracy would be even worse on older systems. Would it be better to use a trigger to snap the player in place maybe?
1 decade ago
by Xatruch
Yea I bet a trigger would be a better solution.
Instead of a trace, why not do this:
//if no collision, move!
if( ig.game.collisionMap.getTile( this.pos.x, this.pos.y + this.size.y + 1 ) === 0 ){
this.vel.x = 0;
this.vel.y = this.speed;
}
1 decade ago
by jul
That's probably a more clever approach to detect the gap quidmonkey, thanks! It will now always detect the gap and apply the y velocity but unfortunately I Still have the same issue where the entity doesn't visit every coordinate. Sometimes it skips the pos.x coordinate where the player lines up with the gap so now it get's stuck instead of continuing along the x axis. At least it's closer to a solution so will experiment some more when I get back from work.
Then lower your vel and floor your pos (and maybe vel) like you&039;ve been doing. If your vel is too high, your Entity will travel more than one pixel per frame. Turn off #.bounciness
if you haven&039;t so that your Entity won't bounce back after colliding with walls. Also, is there some reason you're not using #.handleMovementTrace()
here?
1 decade ago
by jul
Yeah it works flawlessly with lower speed so I'll just do that. Turning off bounciness helped so thanks for that tip too. I've only been using the engine for a couple of days so I'm not sure why I'm not using handleMovementTrace() or how I would benefit from it. Off the read the docs :)
Page 1 of 1
« first
« previous
next ›
last »