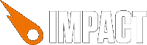
Defined in Module impact.animation, inherits from ig.Class
// Create animation var animSheet = new ig.AnimationSheet( 'sheet.png', 16, 16 ); var anim = new ig.Animation( animSheet, 0.1, [0,1,2,3,2,1] ); // Update animation to current frame anim.update(); // Draw current frame anim.draw( x, y );
An ig.Animation
object takes care of animating an Entity or Background Map tile. Frames from an animation sheet – an image with all animation frames – are drawn as specified by the animations frameTime
and sequence
.
In most cases you shouldn't need to create an animation object by yourself, but instead use the ig.Entity's .addAnim() method.
Read more in the Animations Tutorial.
sheet
An ig.AnimationSheet object specifying the image to use and the width and height of each frameframeTime
Time in seconds each frame is displayedsequence
An Array of integers, specifying the actual animation framesstop
A Boolean, indicating whether to stop the animation when it has reached the last frame. Defaults to false
.Alpha opacity of the animation from 0
to 1
. The default is 1
(fully opaque).
New in 1.15
Rotation of the animation in radians. The center of rotation is specified by .pivot.
Booleans, indicating whether the animation should be drawn flipped on the x and/or y axis
The current frame number, set by the .update()
method
An integer, specifying how often the animation has been played through since the last call to .rewind()
.
Note that even if the animation has stopped at the last frame, the .loopCount
is updated as if the animation was still running.
New in 1.15
Center of the rotation when using .angle. The default is half the .width
and .height
of the AnimationSheet, i.e. the center of the animation frame.
The current tile of the animation sheet, set by the .update()
method
Draws the current animation frame at the given screen coordinates
Jumps to frame number f
Jumps to a random frame
Rewinds the animation to its first frame and resets the .loopCount
This method returns the animation itself. This is useful when switching between animations. E.g:
// In an entities .update() method this.currentAnim = this.anims.jump.rewind();
Updates the animation to the current frame, based on its frameTime